mirror of
https://git.savannah.gnu.org/git/emacs.git
synced 2024-12-01 08:17:38 +00:00
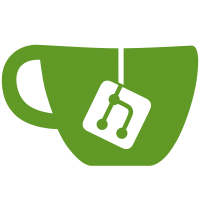
* doc/lispref/os.texi (Desktop Notifications): Document Haiku desktop notifications. * etc/NEWS: Announce this change. * lisp/org/org-clock.el (haiku-notifications-notify): New declaration. (org-show-notification): Employ that function. * src/haiku_io.c (haiku_len) <NOTIFICATION_CLICK_EVENT>: Return the length for this type of event. * src/haiku_select.cc (my_team_id, be_display_notification): New functions. * src/haiku_support.cc (my_team_id, ArgvReceived): New functions. * src/haiku_support.h (enum haiku_event_type): New event type NOTIFICATION_CLICK_EVENT. (struct haiku_notification_click_event): New structure. * src/haikuselect.c (haiku_notifications_notify_1) (Fhaiku_notifications_notify): New functions. (syms_of_haikuselect): Register new defsubr. * src/haikuterm.c (haiku_read_socket): * src/haikuselect.h: * src/termhooks.h: Add new events for notification clicks on Haiku.
981 lines
37 KiB
C
981 lines
37 KiB
C
/* Parameters and display hooks for terminal devices.
|
||
|
||
Copyright (C) 1985-1986, 1993-1994, 2001-2023 Free Software Foundation,
|
||
Inc.
|
||
|
||
This file is part of GNU Emacs.
|
||
|
||
GNU Emacs is free software: you can redistribute it and/or modify
|
||
it under the terms of the GNU General Public License as published by
|
||
the Free Software Foundation, either version 3 of the License, or (at
|
||
your option) any later version.
|
||
|
||
GNU Emacs is distributed in the hope that it will be useful,
|
||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||
GNU General Public License for more details.
|
||
|
||
You should have received a copy of the GNU General Public License
|
||
along with GNU Emacs. If not, see <https://www.gnu.org/licenses/>. */
|
||
|
||
#ifndef EMACS_TERMHOOKS_H
|
||
#define EMACS_TERMHOOKS_H
|
||
|
||
/* Miscellanea. */
|
||
|
||
#include "lisp.h"
|
||
#include "dispextern.h"
|
||
#include "systime.h" /* for Time */
|
||
|
||
struct glyph;
|
||
|
||
INLINE_HEADER_BEGIN
|
||
|
||
enum scroll_bar_part
|
||
{
|
||
scroll_bar_nowhere,
|
||
scroll_bar_above_handle,
|
||
scroll_bar_handle,
|
||
scroll_bar_below_handle,
|
||
scroll_bar_up_arrow,
|
||
scroll_bar_down_arrow,
|
||
scroll_bar_to_top,
|
||
scroll_bar_to_bottom,
|
||
scroll_bar_end_scroll,
|
||
scroll_bar_move_ratio,
|
||
scroll_bar_before_handle,
|
||
scroll_bar_horizontal_handle,
|
||
scroll_bar_after_handle,
|
||
scroll_bar_left_arrow,
|
||
scroll_bar_right_arrow,
|
||
scroll_bar_to_leftmost,
|
||
scroll_bar_to_rightmost
|
||
};
|
||
|
||
/* Output method of a terminal (and frames on this terminal, respectively). */
|
||
|
||
enum output_method
|
||
{
|
||
output_initial,
|
||
output_termcap,
|
||
output_x_window,
|
||
output_msdos_raw,
|
||
output_w32,
|
||
output_ns,
|
||
output_pgtk,
|
||
output_haiku,
|
||
output_android,
|
||
};
|
||
|
||
/* Input queue declarations and hooks. */
|
||
|
||
enum event_kind
|
||
{
|
||
NO_EVENT, /* nothing happened. This should never
|
||
actually appear in the event queue. */
|
||
|
||
ASCII_KEYSTROKE_EVENT, /* The ASCII code is in .code, perhaps
|
||
with modifiers applied.
|
||
.modifiers holds the state of the
|
||
modifier keys.
|
||
.frame_or_window is the frame in
|
||
which the key was typed.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the keystroke. */
|
||
MULTIBYTE_CHAR_KEYSTROKE_EVENT, /* The multibyte char code is
|
||
in .code, perhaps with
|
||
modifiers applied. The
|
||
others are the same as
|
||
ASCII_KEYSTROKE_EVENT,
|
||
except when ARG is a
|
||
string, which will be
|
||
decoded and the decoded
|
||
string's characters will be
|
||
used as .code
|
||
individually.
|
||
|
||
The string can have a
|
||
property `coding', which
|
||
should be a symbol
|
||
describing a coding system
|
||
to use to decode the string.
|
||
|
||
If it is nil, then the
|
||
locale coding system will
|
||
be used. If it is t, then
|
||
no decoding will take
|
||
place. */
|
||
NON_ASCII_KEYSTROKE_EVENT, /* .code is a number identifying the
|
||
function key. A code N represents
|
||
a key whose name is
|
||
function_key_names[N]; function_key_names
|
||
is a table in keyboard.c to which you
|
||
should feel free to add missing keys.
|
||
.modifiers holds the state of the
|
||
modifier keys.
|
||
.frame_or_window is the frame in
|
||
which the key was typed.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the keystroke. */
|
||
TIMER_EVENT, /* A timer fired. */
|
||
MOUSE_CLICK_EVENT, /* The button number is in .code; it must
|
||
be >= 0 and < NUM_MOUSE_BUTTONS, defined
|
||
below.
|
||
.modifiers holds the state of the
|
||
modifier keys.
|
||
.x and .y give the mouse position,
|
||
in characters, within the window.
|
||
.frame_or_window gives the frame
|
||
the mouse click occurred in.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the click. */
|
||
WHEEL_EVENT, /* A wheel event is generated by a
|
||
wheel on a mouse (e.g., MS
|
||
Intellimouse).
|
||
.modifiers holds the rotate
|
||
direction (up or down), and the
|
||
state of the modifier keys.
|
||
.x and .y give the mouse position,
|
||
in characters, within the window.
|
||
.frame_or_window gives the frame
|
||
the wheel event occurred in.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the event.
|
||
.arg may contain the number of
|
||
lines to scroll, or a list of
|
||
the form (NUMBER-OF-LINES . (X Y)) where
|
||
X and Y are the number of pixels
|
||
on each axis to scroll by. */
|
||
HORIZ_WHEEL_EVENT, /* A wheel event generated by a second
|
||
horizontal wheel that is present on some
|
||
mice. See WHEEL_EVENT. */
|
||
#ifdef HAVE_NTGUI
|
||
LANGUAGE_CHANGE_EVENT, /* A LANGUAGE_CHANGE_EVENT is
|
||
generated when HAVE_NTGUI or on Mac OS
|
||
when the keyboard layout or input
|
||
language is changed by the
|
||
user. */
|
||
#endif
|
||
SCROLL_BAR_CLICK_EVENT, /* .code gives the number of the mouse button
|
||
that was clicked.
|
||
.modifiers holds the state of the modifier
|
||
keys.
|
||
.part is a lisp symbol indicating which
|
||
part of the scroll bar got clicked.
|
||
.x gives the distance from the start of the
|
||
scroll bar of the click; .y gives the total
|
||
length of the scroll bar.
|
||
.frame_or_window gives the window
|
||
whose scroll bar was clicked in.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the click. */
|
||
HORIZONTAL_SCROLL_BAR_CLICK_EVENT, /* .code gives the number of the mouse button
|
||
that was clicked.
|
||
.modifiers holds the state of the modifier
|
||
keys.
|
||
.part is a lisp symbol indicating which
|
||
part of the scroll bar got clicked.
|
||
.x gives the distance from the start of the
|
||
scroll bar of the click; .y gives the total
|
||
length of the scroll bar.
|
||
.frame_or_window gives the window
|
||
whose scroll bar was clicked in.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the click. */
|
||
SELECTION_REQUEST_EVENT, /* Another X client wants a selection from us.
|
||
See `struct selection_input_event'. */
|
||
SELECTION_CLEAR_EVENT, /* Another X client cleared our selection. */
|
||
DELETE_WINDOW_EVENT, /* An X client said "delete this window". */
|
||
#ifdef HAVE_NTGUI
|
||
END_SESSION_EVENT, /* The user is logging out or shutting down. */
|
||
#endif
|
||
MENU_BAR_EVENT, /* An event generated by the menu bar.
|
||
The frame_or_window field's cdr holds the
|
||
Lisp-level event value.
|
||
(Only the toolkit version uses these.) */
|
||
ICONIFY_EVENT, /* An X client iconified this window. */
|
||
DEICONIFY_EVENT, /* An X client deiconified this window. */
|
||
MENU_BAR_ACTIVATE_EVENT, /* A button press in the menu bar
|
||
(toolkit version only). */
|
||
DRAG_N_DROP_EVENT, /* A drag-n-drop event is generated when
|
||
files selected outside of Emacs are dropped
|
||
onto an Emacs window.
|
||
.modifiers holds the state of the
|
||
modifier keys.
|
||
.x and .y give the mouse position,
|
||
in characters, within the window.
|
||
.frame_or_window is the frame in
|
||
which the drop was made.
|
||
.arg is a platform-dependent
|
||
representation of the dropped items.
|
||
.timestamp gives a timestamp (in
|
||
milliseconds) for the click. */
|
||
USER_SIGNAL_EVENT, /* A user signal.
|
||
code is a number identifying it,
|
||
index into lispy_user_signals. */
|
||
|
||
/* Help events. Member `frame_or_window' of the input_event is the
|
||
frame on which the event occurred, and member `arg' contains
|
||
the help to show. */
|
||
HELP_EVENT,
|
||
|
||
/* An event from a tab-bar. Member `arg' of the input event
|
||
contains the tab-bar item selected. If `frame_or_window'
|
||
and `arg' are equal, this is a prefix event. */
|
||
TAB_BAR_EVENT,
|
||
|
||
/* An event from a tool-bar. Member `arg' of the input event
|
||
contains the tool-bar item selected. If `frame_or_window'
|
||
and `arg' are equal, this is a prefix event. */
|
||
TOOL_BAR_EVENT,
|
||
|
||
/* Queued from XTread_socket on FocusIn events. Translated into
|
||
`switch-frame' events in kbd_buffer_get_event, if necessary. */
|
||
FOCUS_IN_EVENT,
|
||
|
||
FOCUS_OUT_EVENT,
|
||
|
||
/* Generated when a frame is moved. */
|
||
MOVE_FRAME_EVENT,
|
||
|
||
/* Generated when mouse moves over window not currently selected. */
|
||
SELECT_WINDOW_EVENT,
|
||
|
||
/* Queued from XTread_socket when session manager sends
|
||
save yourself before shutdown. */
|
||
SAVE_SESSION_EVENT
|
||
|
||
#ifdef HAVE_DBUS
|
||
, DBUS_EVENT
|
||
#endif
|
||
|
||
#ifdef THREADS_ENABLED
|
||
, THREAD_EVENT
|
||
#endif
|
||
|
||
, CONFIG_CHANGED_EVENT
|
||
|
||
#ifdef HAVE_NTGUI
|
||
/* Generated when an APPCOMMAND event is received, in response to
|
||
Multimedia or Internet buttons on some keyboards.
|
||
Such keys are available as normal function keys on X through the
|
||
Xkeyboard extension.
|
||
On Windows, some of them get mapped to normal function key events,
|
||
but others need to be handled by APPCOMMAND. Handling them all as
|
||
APPCOMMAND events means they can be disabled
|
||
(w32-pass-multimedia-buttons-to-system), important on Windows since
|
||
the system never sees these keys if Emacs claims to handle them.
|
||
On X, the window manager seems to grab the keys it wants
|
||
first, so this is not a problem there. */
|
||
, MULTIMEDIA_KEY_EVENT
|
||
#endif
|
||
|
||
#ifdef HAVE_NS
|
||
/* Generated when native multi-keystroke input method is used to modify
|
||
tentative or indicative text display. */
|
||
, NS_TEXT_EVENT
|
||
/* Non-key system events (e.g. application menu events) */
|
||
, NS_NONKEY_EVENT
|
||
#endif
|
||
|
||
#ifdef HAVE_XWIDGETS
|
||
/* An event generated by an xwidget to tell us something. */
|
||
, XWIDGET_EVENT
|
||
|
||
/* Event generated when WebKit asks us to display another widget. */
|
||
, XWIDGET_DISPLAY_EVENT
|
||
#endif
|
||
|
||
#ifdef USE_FILE_NOTIFY
|
||
/* File or directory was changed. */
|
||
, FILE_NOTIFY_EVENT
|
||
#endif
|
||
|
||
/* Pre-edit text was changed. */
|
||
, PREEDIT_TEXT_EVENT
|
||
|
||
/* Either the mouse wheel has been released without it being
|
||
clicked, or the user has lifted his finger from a touchpad.
|
||
|
||
In the future, this may take into account other multi-touch
|
||
events generated from touchscreens and such. */
|
||
, TOUCH_END_EVENT
|
||
|
||
/* In a TOUCHSCREEN_UPDATE_EVENT, ARG is a list of elements of the
|
||
form (X Y ID), where X and Y are the coordinates of the
|
||
touchpoint relative to the top-left corner of the frame, and ID
|
||
is a unique number identifying the touchpoint.
|
||
|
||
In TOUCHSCREEN_BEGIN_EVENT and TOUCHSCREEN_END_EVENT, ARG is the
|
||
unique ID of the touchpoint, and X and Y are the frame-relative
|
||
positions of the touchpoint.
|
||
|
||
In TOUCHSCREEN_END_EVENT, non-0 modifiers means that the
|
||
touchpoint has been canceled. (See (elisp)Touchscreen
|
||
Events.) */
|
||
|
||
, TOUCHSCREEN_UPDATE_EVENT
|
||
, TOUCHSCREEN_BEGIN_EVENT
|
||
, TOUCHSCREEN_END_EVENT
|
||
|
||
/* In a PINCH_EVENT, X and Y are the position of the pointer
|
||
relative to the top-left corner of the frame, and arg is a list
|
||
of (DX DY SCALE ANGLE), in which:
|
||
|
||
- DX and DY are the difference between the positions of the
|
||
fingers comprising the current gesture and the last such
|
||
gesture in the same sequence.
|
||
- SCALE is the division of the current distance between the
|
||
fingers and the distance at the start of the gesture.
|
||
- DELTA-ANGLE is the delta between the angle of the current
|
||
event and the last event in the same sequence, in degrees. A
|
||
positive delta represents a change clockwise, and a negative
|
||
delta represents a change counter-clockwise. */
|
||
, PINCH_EVENT
|
||
|
||
/* In a MONITORS_CHANGED_EVENT, .arg gives the terminal on which the
|
||
monitor configuration changed. .timestamp gives the time on
|
||
which the monitors changed. */
|
||
, MONITORS_CHANGED_EVENT
|
||
|
||
#ifdef HAVE_HAIKU
|
||
/* In a NOTIFICATION_CLICKED_EVENT, .arg is an integer identifying
|
||
the notification that was clicked. */
|
||
, NOTIFICATION_CLICKED_EVENT
|
||
#endif /* HAVE_HAIKU */
|
||
};
|
||
|
||
/* Bit width of an enum event_kind tag at the start of structs and unions. */
|
||
enum { EVENT_KIND_WIDTH = 16 };
|
||
|
||
/* If a struct input_event has a kind which is SELECTION_REQUEST_EVENT
|
||
or SELECTION_CLEAR_EVENT, then its contents are really described
|
||
by `struct selection_input_event'; see xterm.h. */
|
||
|
||
/* The keyboard input buffer is an array of these structures. Each one
|
||
represents some sort of input event - a keystroke, a mouse click, or
|
||
a window system event. These get turned into their lispy forms when
|
||
they are removed from the event queue. */
|
||
|
||
struct input_event
|
||
{
|
||
/* What kind of event was this? */
|
||
ENUM_BF (event_kind) kind : EVENT_KIND_WIDTH;
|
||
|
||
/* Used in scroll back click events. */
|
||
ENUM_BF (scroll_bar_part) part : 16;
|
||
|
||
/* For an ASCII_KEYSTROKE_EVENT and MULTIBYTE_CHAR_KEYSTROKE_EVENT,
|
||
this is the character.
|
||
For a NON_ASCII_KEYSTROKE_EVENT, this is the keysym code.
|
||
For a mouse event, this is the button number. */
|
||
unsigned code;
|
||
|
||
/* See enum below for interpretation. */
|
||
unsigned modifiers;
|
||
|
||
/* One would prefer C integers, but HELP_EVENT uses these to
|
||
record frame or window object and a help form, respectively. */
|
||
Lisp_Object x, y;
|
||
|
||
/* Usually a time as reported by window system-specific event loop.
|
||
For a HELP_EVENT, this is the position within the object (stored
|
||
in ARG below) where the help was found. */
|
||
Time timestamp;
|
||
|
||
/* This field is copied into a vector while the event is in
|
||
the queue, so that garbage collections won't kill it. */
|
||
Lisp_Object frame_or_window;
|
||
|
||
/* This additional argument is used in attempt to avoid extra consing
|
||
when building events. Unfortunately some events have to pass much
|
||
more data than it's reasonable to pack directly into this structure. */
|
||
Lisp_Object arg;
|
||
|
||
/* The name of the device from which this event originated.
|
||
|
||
It can either be a string, or Qt, which means to use the name
|
||
"Virtual core pointer" for all events other than keystroke
|
||
events, and "Virtual core keyboard" for those. */
|
||
Lisp_Object device;
|
||
};
|
||
|
||
#define EVENT_INIT(event) (memset (&(event), 0, sizeof (struct input_event)), \
|
||
(event).device = Qt)
|
||
|
||
/* Bits in the modifiers member of the input_event structure.
|
||
Note that reorder_modifiers assumes that the bits are in canonical
|
||
order.
|
||
|
||
The modifiers applied to mouse clicks are rather ornate. The
|
||
window-system-specific code should store mouse clicks with
|
||
up_modifier or down_modifier set. Having an explicit down modifier
|
||
simplifies some of window-system-independent code; without it, the
|
||
code would have to recognize down events by checking if the event
|
||
is a mouse click lacking the click and drag modifiers.
|
||
|
||
The window-system independent code turns all up_modifier events
|
||
bits into drag_modifier, click_modifier, double_modifier, or
|
||
triple_modifier events. The click_modifier has no written
|
||
representation in the names of the symbols used as event heads,
|
||
but it does appear in the Qevent_symbol_components property of the
|
||
event heads. */
|
||
enum {
|
||
up_modifier = 1, /* Only used on mouse buttons - always
|
||
turned into a click or a drag modifier
|
||
before lisp code sees the event. */
|
||
down_modifier = 2, /* Only used on mouse buttons. */
|
||
drag_modifier = 4, /* This is never used in the event
|
||
queue; it's only used internally by
|
||
the window-system-independent code. */
|
||
click_modifier= 8, /* See drag_modifier. */
|
||
double_modifier= 16, /* See drag_modifier. */
|
||
triple_modifier= 32, /* See drag_modifier. */
|
||
|
||
/* The next four modifier bits are used also in keyboard events at
|
||
the Lisp level.
|
||
|
||
It's probably not the greatest idea to use the 2^28 bit for any
|
||
modifier. It may or may not be the sign bit, depending on
|
||
FIXNUM_BITS, so using it to represent a modifier key means that
|
||
characters thus modified have different integer equivalents
|
||
depending on the architecture they're running on. Oh, and
|
||
applying XFIXNUM to a character whose 2^28 bit is set might sign-extend
|
||
it, so you get a bunch of bits in the mask you didn't want.
|
||
|
||
The CHAR_ macros are defined in lisp.h. */
|
||
alt_modifier = CHAR_ALT, /* Under X, the XK_Alt_[LR] keysyms. */
|
||
super_modifier= CHAR_SUPER, /* Under X, the XK_Super_[LR] keysyms. */
|
||
hyper_modifier= CHAR_HYPER, /* Under X, the XK_Hyper_[LR] keysyms. */
|
||
shift_modifier= CHAR_SHIFT,
|
||
ctrl_modifier = CHAR_CTL,
|
||
meta_modifier = CHAR_META /* Under X, the XK_Meta_[LR] keysyms. */
|
||
};
|
||
|
||
#ifdef HAVE_GPM
|
||
#include <gpm.h>
|
||
extern int handle_one_term_event (struct tty_display_info *, Gpm_Event *);
|
||
extern void term_mouse_moveto (int, int);
|
||
|
||
/* The device for which we have enabled gpm support. */
|
||
extern struct tty_display_info *gpm_tty;
|
||
#endif
|
||
|
||
/* Terminal-local parameters. */
|
||
struct terminal
|
||
{
|
||
/* This is for Lisp; the terminal code does not refer to it. */
|
||
union vectorlike_header header;
|
||
|
||
/* Parameter alist of this terminal. */
|
||
Lisp_Object param_alist;
|
||
|
||
/* List of charsets supported by the terminal. It is set by
|
||
Fset_terminal_coding_system_internal along with
|
||
the member terminal_coding. */
|
||
Lisp_Object charset_list;
|
||
|
||
/* This is an association list containing the X selections that
|
||
Emacs might own on this terminal. Each element has the form
|
||
(SELECTION-NAME SELECTION-VALUE SELECTION-TIMESTAMP FRAME)
|
||
SELECTION-NAME is a lisp symbol, whose name is the name of an X Atom.
|
||
SELECTION-VALUE is the value that emacs owns for that selection.
|
||
It may be any kind of Lisp object.
|
||
SELECTION-TIMESTAMP is the time at which emacs began owning this
|
||
selection, as a cons of two 16-bit numbers (making a 32 bit
|
||
time.)
|
||
FRAME is the frame for which we made the selection. If there is
|
||
an entry in this alist, then it can be assumed that Emacs owns
|
||
that selection.
|
||
The only (eq) parts of this list that are visible from Lisp are
|
||
the selection-values. */
|
||
Lisp_Object Vselection_alist;
|
||
|
||
/* If a char-table, this maps characters to terminal glyph codes.
|
||
If t, the mapping is not available. If nil, it is not known
|
||
whether the mapping is available. */
|
||
Lisp_Object glyph_code_table;
|
||
|
||
/* All earlier fields should be Lisp_Objects and are traced
|
||
by the GC. All fields afterwards are ignored by the GC. */
|
||
|
||
/* Chain of all terminal devices. */
|
||
struct terminal *next_terminal;
|
||
|
||
/* Unique id for this terminal device. */
|
||
int id;
|
||
|
||
/* The number of frames that are on this terminal. */
|
||
int reference_count;
|
||
|
||
/* The type of the terminal device. */
|
||
enum output_method type;
|
||
|
||
/* The name of the terminal device. Do not use this to uniquely
|
||
identify a terminal; the same device may be opened multiple
|
||
times. */
|
||
char *name;
|
||
|
||
/* The terminal's keyboard object. */
|
||
struct kboard *kboard;
|
||
|
||
#ifdef HAVE_WINDOW_SYSTEM
|
||
/* Cache of images. */
|
||
struct image_cache *image_cache;
|
||
#endif /* HAVE_WINDOW_SYSTEM */
|
||
|
||
/* Device-type dependent data shared amongst all frames on this terminal. */
|
||
union display_info
|
||
{
|
||
struct tty_display_info *tty; /* termchar.h */
|
||
struct x_display_info *x; /* xterm.h */
|
||
struct w32_display_info *w32; /* w32term.h */
|
||
struct ns_display_info *ns; /* nsterm.h */
|
||
struct pgtk_display_info *pgtk; /* pgtkterm.h */
|
||
struct haiku_display_info *haiku; /* haikuterm.h */
|
||
struct android_display_info *android; /* androidterm.h */
|
||
} display_info;
|
||
|
||
|
||
/* Coding-system to be used for encoding terminal output. This
|
||
structure contains information of a coding-system specified by
|
||
the function `set-terminal-coding-system'. Also see
|
||
`safe_terminal_coding' in coding.h. */
|
||
struct coding_system *terminal_coding;
|
||
|
||
/* Coding-system of what is sent from terminal keyboard. This
|
||
structure contains information of a coding-system specified by
|
||
the function `set-keyboard-coding-system'. */
|
||
struct coding_system *keyboard_coding;
|
||
|
||
/* Window-based redisplay interface for this device (0 for tty
|
||
devices). */
|
||
struct redisplay_interface *rif;
|
||
|
||
/* Frame-based redisplay interface. */
|
||
|
||
/* Text display hooks. */
|
||
|
||
void (*cursor_to_hook) (struct frame *f, int vpos, int hpos);
|
||
void (*raw_cursor_to_hook) (struct frame *, int, int);
|
||
|
||
void (*clear_to_end_hook) (struct frame *);
|
||
void (*clear_frame_hook) (struct frame *);
|
||
void (*clear_end_of_line_hook) (struct frame *, int);
|
||
|
||
void (*ins_del_lines_hook) (struct frame *f, int, int);
|
||
|
||
void (*insert_glyphs_hook) (struct frame *f, struct glyph *s, int n);
|
||
void (*write_glyphs_hook) (struct frame *f, struct glyph *s, int n);
|
||
void (*delete_glyphs_hook) (struct frame *, int);
|
||
|
||
void (*ring_bell_hook) (struct frame *f);
|
||
void (*toggle_invisible_pointer_hook) (struct frame *f, bool invisible);
|
||
|
||
void (*reset_terminal_modes_hook) (struct terminal *);
|
||
void (*set_terminal_modes_hook) (struct terminal *);
|
||
|
||
void (*update_begin_hook) (struct frame *);
|
||
void (*update_end_hook) (struct frame *);
|
||
void (*set_terminal_window_hook) (struct frame *, int);
|
||
|
||
/* Decide if color named COLOR_NAME is valid for the display
|
||
associated with the frame F; if so, return the RGB values in
|
||
COLOR_DEF. If ALLOC (and MAKEINDEX for NS), allocate a new
|
||
colormap cell.
|
||
|
||
If MAKEINDEX (on NS), set COLOR_DEF pixel to ARGB. */
|
||
bool (*defined_color_hook) (struct frame *f, const char *color_name,
|
||
Emacs_Color *color_def,
|
||
bool alloc,
|
||
bool makeIndex);
|
||
|
||
/* Multi-frame and mouse support hooks. */
|
||
|
||
/* Graphical window systems are expected to define all of the
|
||
following hooks with the possible exception of:
|
||
|
||
* query_colors
|
||
* activate_menubar_hook
|
||
* change_tool_bar_height_hook
|
||
* set_bitmap_icon_hook
|
||
* buffer_flipping_unblocked_hook
|
||
|
||
*/
|
||
|
||
/* This hook is called to store the frame's background color into
|
||
BGCOLOR. */
|
||
void (*query_frame_background_color) (struct frame *f, Emacs_Color *bgcolor);
|
||
|
||
#if defined (HAVE_X_WINDOWS) || defined (HAVE_NTGUI) || defined (HAVE_PGTK) \
|
||
|| defined (HAVE_ANDROID)
|
||
/* On frame F, translate pixel colors to RGB values for the NCOLORS
|
||
colors in COLORS. Use cached information, if available. */
|
||
|
||
void (*query_colors) (struct frame *f, Emacs_Color *colors, int ncolors);
|
||
#endif
|
||
/* Return the current position of the mouse.
|
||
|
||
Set *f to the frame the mouse is in, or zero if the mouse is in no
|
||
Emacs frame. If it is set to zero, all the other arguments are
|
||
garbage.
|
||
|
||
If the motion started in a scroll bar, set *bar_window to the
|
||
scroll bar's window, *part to the part the mouse is currently over,
|
||
*x to the position of the mouse along the scroll bar, and *y to the
|
||
overall length of the scroll bar.
|
||
|
||
Otherwise, set *bar_window to Qnil, and *x and *y to the column and
|
||
row of the character cell the mouse is over.
|
||
|
||
Set *time to the time the mouse was at the returned position. */
|
||
void (*mouse_position_hook) (struct frame **f, int,
|
||
Lisp_Object *bar_window,
|
||
enum scroll_bar_part *part,
|
||
Lisp_Object *x,
|
||
Lisp_Object *y,
|
||
Time *);
|
||
|
||
/* This hook is called to get the focus frame. */
|
||
Lisp_Object (*get_focus_frame) (struct frame *f);
|
||
|
||
/* This hook is called to shift frame focus. */
|
||
void (*focus_frame_hook) (struct frame *f, bool noactivate);
|
||
|
||
/* When a frame's focus redirection is changed, this hook tells the
|
||
window system code to re-decide where to put the highlight. Under
|
||
X, this means that Emacs lies about where the focus is. */
|
||
void (*frame_rehighlight_hook) (struct frame *);
|
||
|
||
/* If we're displaying frames using a window system that can stack
|
||
frames on top of each other, this hook allows you to bring a frame
|
||
to the front, or bury it behind all the other windows. If this
|
||
hook is zero, that means the terminal we're displaying on doesn't
|
||
support overlapping frames, so there's no need to raise or lower
|
||
anything.
|
||
|
||
If RAISE_FLAG, F is brought to the front, before all other
|
||
windows. If !RAISE_FLAG, F is sent to the back, behind all other
|
||
windows. */
|
||
void (*frame_raise_lower_hook) (struct frame *f, bool raise_flag);
|
||
|
||
/* This hook is called to make the frame F visible if VISIBLE is
|
||
true, or invisible otherwise. */
|
||
void (*frame_visible_invisible_hook) (struct frame *f, bool visible);
|
||
|
||
/* If the value of the frame parameter changed, this hook is called.
|
||
For example, if going from fullscreen to not fullscreen this hook
|
||
may do something OS dependent, like extended window manager hints on X11. */
|
||
void (*fullscreen_hook) (struct frame *f);
|
||
|
||
/* This hook is called to iconify the frame. */
|
||
void (*iconify_frame_hook) (struct frame *f);
|
||
|
||
/* This hook is called to change the size of frame F's native
|
||
(underlying) window. If CHANGE_GRAVITY, change to top-left-corner
|
||
window gravity for this size change and subsequent size changes.
|
||
Otherwise we leave the window gravity unchanged. */
|
||
void (*set_window_size_hook) (struct frame *f, bool change_gravity,
|
||
int width, int height);
|
||
|
||
/* CHANGE_GRAVITY is 1 when calling from Fset_frame_position,
|
||
to really change the position, and 0 when calling from
|
||
*_make_frame_visible (in that case, XOFF and YOFF are the current
|
||
position values). It is -1 when calling from gui_set_frame_parameters,
|
||
which means, do adjust for borders but don't change the gravity. */
|
||
|
||
void (*set_frame_offset_hook) (struct frame *f, register int xoff,
|
||
register int yoff, int change_gravity);
|
||
|
||
/* This hook is called to set the frame's transparency. */
|
||
void (*set_frame_alpha_hook) (struct frame *f);
|
||
|
||
/* This hook is called to set a new font for the frame. */
|
||
Lisp_Object (*set_new_font_hook) (struct frame *f, Lisp_Object font_object,
|
||
int fontset);
|
||
|
||
/* This hook is called to set the GUI window icon of F using FILE. */
|
||
bool (*set_bitmap_icon_hook) (struct frame *f, Lisp_Object file);
|
||
|
||
/* This hook is called to set the name of the GUI window of F by
|
||
redisplay unless another name was explicitly requested. */
|
||
void (*implicit_set_name_hook) (struct frame *f, Lisp_Object arg,
|
||
Lisp_Object oldval);
|
||
|
||
/* This hook is called to display menus. */
|
||
Lisp_Object (*menu_show_hook) (struct frame *f, int x, int y, int menuflags,
|
||
Lisp_Object title, const char **error_name);
|
||
|
||
#ifdef HAVE_EXT_MENU_BAR
|
||
/* This hook is called to activate the menu bar. */
|
||
void (*activate_menubar_hook) (struct frame *f);
|
||
#endif
|
||
|
||
/* This hook is called to display popup dialog. */
|
||
Lisp_Object (*popup_dialog_hook) (struct frame *f, Lisp_Object header,
|
||
Lisp_Object contents);
|
||
|
||
/* This hook is called to change the frame's (internal) tab-bar. */
|
||
void (*change_tab_bar_height_hook) (struct frame *f, int height);
|
||
|
||
/* This hook is called to change the frame's (internal) tool-bar. */
|
||
void (*change_tool_bar_height_hook) (struct frame *f, int height);
|
||
|
||
/* Scroll bar hooks. */
|
||
|
||
/* The representation of scroll bars is determined by the code which
|
||
implements them, except for one thing: they must be represented by
|
||
lisp objects. This allows us to place references to them in
|
||
Lisp_Windows without worrying about those references becoming
|
||
dangling references when the scroll bar is destroyed.
|
||
|
||
The window-system-independent portion of Emacs just refers to
|
||
scroll bars via their windows, and never looks inside the scroll bar
|
||
representation; it always uses hook functions to do all the
|
||
scroll bar manipulation it needs.
|
||
|
||
The `vertical_scroll_bar' field of a Lisp_Window refers to that
|
||
window's scroll bar, or is nil if the window doesn't have a
|
||
scroll bar.
|
||
|
||
The `scroll_bars' and `condemned_scroll_bars' fields of a Lisp_Frame
|
||
are free for use by the scroll bar implementation in any way it sees
|
||
fit. They are marked by the garbage collector. */
|
||
|
||
|
||
/* Set the vertical scroll bar for WINDOW to have its upper left corner
|
||
at (TOP, LEFT), and be LENGTH rows high. Set its handle to
|
||
indicate that we are displaying PORTION characters out of a total
|
||
of WHOLE characters, starting at POSITION. If WINDOW doesn't yet
|
||
have a scroll bar, create one for it. */
|
||
void (*set_vertical_scroll_bar_hook) (struct window *window,
|
||
int portion, int whole,
|
||
int position);
|
||
|
||
|
||
/* Set the horizontal scroll bar for WINDOW to have its upper left
|
||
corner at (TOP, LEFT), and be LENGTH rows high. Set its handle to
|
||
indicate that we are displaying PORTION characters out of a total
|
||
of WHOLE characters, starting at POSITION. If WINDOW doesn't yet
|
||
have a scroll bar, create one for it. */
|
||
void (*set_horizontal_scroll_bar_hook) (struct window *window,
|
||
int portion, int whole,
|
||
int position);
|
||
|
||
/* Set the default scroll bar width on FRAME. */
|
||
void (*set_scroll_bar_default_width_hook) (struct frame *frame);
|
||
|
||
/* Set the default scroll bar height on FRAME. */
|
||
void (*set_scroll_bar_default_height_hook) (struct frame *frame);
|
||
|
||
/* The following three hooks are used when we're doing a thorough
|
||
redisplay of the frame. We don't explicitly know which scroll bars
|
||
are going to be deleted, because keeping track of when windows go
|
||
away is a real pain - can you say set-window-configuration?
|
||
Instead, we just assert at the beginning of redisplay that *all*
|
||
scroll bars are to be removed, and then save scroll bars from the
|
||
fiery pit when we actually redisplay their window. */
|
||
|
||
/* Arrange for all scroll bars on FRAME to be removed at the next call
|
||
to `*judge_scroll_bars_hook'. A scroll bar may be spared if
|
||
`*redeem_scroll_bar_hook' is applied to its window before the judgment.
|
||
|
||
This should be applied to each frame each time its window tree is
|
||
redisplayed, even if it is not displaying scroll bars at the moment;
|
||
if the HAS_SCROLL_BARS flag has just been turned off, only calling
|
||
this and the judge_scroll_bars_hook will get rid of them.
|
||
|
||
If non-zero, this hook should be safe to apply to any frame,
|
||
whether or not it can support scroll bars, and whether or not it is
|
||
currently displaying them. */
|
||
void (*condemn_scroll_bars_hook) (struct frame *frame);
|
||
|
||
/* Unmark WINDOW's scroll bar for deletion in this judgment cycle.
|
||
Note that it's okay to redeem a scroll bar that is not condemned. */
|
||
void (*redeem_scroll_bar_hook) (struct window *window);
|
||
|
||
/* Remove all scroll bars on FRAME that haven't been saved since the
|
||
last call to `*condemn_scroll_bars_hook'.
|
||
|
||
This should be applied to each frame after each time its window
|
||
tree is redisplayed, even if it is not displaying scroll bars at the
|
||
moment; if the HAS_SCROLL_BARS flag has just been turned off, only
|
||
calling this and condemn_scroll_bars_hook will get rid of them.
|
||
|
||
If non-zero, this hook should be safe to apply to any frame,
|
||
whether or not it can support scroll bars, and whether or not it is
|
||
currently displaying them. */
|
||
void (*judge_scroll_bars_hook) (struct frame *FRAME);
|
||
|
||
|
||
/* Called to read input events.
|
||
|
||
TERMINAL indicates which terminal device to read from. Input
|
||
events should be read into HOLD_QUIT.
|
||
|
||
A positive return value N indicates that N input events
|
||
were read into BUF.
|
||
Zero means no events were immediately available.
|
||
A value of -1 means a transient read error, while -2 indicates
|
||
that the device was closed (hangup), and it should be deleted. */
|
||
int (*read_socket_hook) (struct terminal *terminal,
|
||
struct input_event *hold_quit);
|
||
|
||
/* Called when a frame's display becomes entirely up to date. */
|
||
void (*frame_up_to_date_hook) (struct frame *);
|
||
|
||
/* Called when buffer flipping becomes unblocked after having
|
||
previously been blocked. Redisplay always blocks buffer flips
|
||
while it runs. */
|
||
void (*buffer_flipping_unblocked_hook) (struct frame *);
|
||
|
||
/* Retrieve the string resource specified by NAME with CLASS from
|
||
database RDB. */
|
||
const char * (*get_string_resource_hook) (void *rdb,
|
||
const char *name,
|
||
const char *class);
|
||
|
||
/* Image hooks */
|
||
#ifdef HAVE_WINDOW_SYSTEM
|
||
/* Free the pixmap PIXMAP on F. */
|
||
void (*free_pixmap) (struct frame *f, Emacs_Pixmap pixmap);
|
||
|
||
#endif
|
||
|
||
/* Deletion hooks */
|
||
|
||
/* Called to delete the device-specific portions of a frame that is
|
||
on this terminal device. */
|
||
void (*delete_frame_hook) (struct frame *);
|
||
|
||
/* Called after the last frame on this terminal is deleted, or when
|
||
the display device was closed (hangup).
|
||
|
||
If this is NULL, then the generic delete_terminal is called
|
||
instead. Otherwise the hook must call delete_terminal itself.
|
||
|
||
The hook must check for and close any live frames that are still
|
||
on the terminal. delete_frame ensures that there are no live
|
||
frames on the terminal when it calls this hook, so infinite
|
||
recursion is prevented. */
|
||
void (*delete_terminal_hook) (struct terminal *);
|
||
|
||
/* Called to determine whether a position is on the toolkit tool bar
|
||
or menu bar. May be NULL. It should accept five arguments
|
||
FRAME, X, Y, MENU_BAR_P, TOOL_BAR_P, and store true into
|
||
MENU_BAR_P if X and Y are in FRAME's toolkit menu bar, and true
|
||
into TOOL_BAR_P if X and Y are in FRAME's toolkit tool bar. */
|
||
void (*toolkit_position_hook) (struct frame *, int, int, bool *, bool *);
|
||
|
||
#ifdef HAVE_WINDOW_SYSTEM
|
||
/* Called to determine if the mouse is grabbed on the given display.
|
||
If either dpyinfo->grabbed or this returns true, then the display
|
||
will be considered as grabbed. */
|
||
bool (*any_grab_hook) (Display_Info *);
|
||
#endif
|
||
} GCALIGNED_STRUCT;
|
||
|
||
INLINE bool
|
||
TERMINALP (Lisp_Object a)
|
||
{
|
||
return PSEUDOVECTORP (a, PVEC_TERMINAL);
|
||
}
|
||
|
||
INLINE struct terminal *
|
||
XTERMINAL (Lisp_Object a)
|
||
{
|
||
eassert (TERMINALP (a));
|
||
return XUNTAG (a, Lisp_Vectorlike, struct terminal);
|
||
}
|
||
|
||
/* Most code should use these functions to set Lisp fields in struct
|
||
terminal. */
|
||
INLINE void
|
||
tset_charset_list (struct terminal *t, Lisp_Object val)
|
||
{
|
||
t->charset_list = val;
|
||
}
|
||
INLINE void
|
||
tset_selection_alist (struct terminal *t, Lisp_Object val)
|
||
{
|
||
t->Vselection_alist = val;
|
||
}
|
||
|
||
/* Chain of all terminal devices currently in use. */
|
||
extern struct terminal *terminal_list;
|
||
|
||
#define FRAME_MUST_WRITE_SPACES(f) (FRAME_TTY (f)->must_write_spaces)
|
||
#define FRAME_LINE_INS_DEL_OK(f) (FRAME_TTY (f)->line_ins_del_ok)
|
||
#define FRAME_CHAR_INS_DEL_OK(f) (FRAME_TTY (f)->char_ins_del_ok)
|
||
#define FRAME_SCROLL_REGION_OK(f) (FRAME_TTY (f)->scroll_region_ok)
|
||
#define FRAME_SCROLL_REGION_COST(f) (FRAME_TTY (f)->scroll_region_cost)
|
||
#define FRAME_MEMORY_BELOW_FRAME(f) (FRAME_TTY (f)->memory_below_frame)
|
||
|
||
#define FRAME_TERMINAL_CODING(f) ((f)->terminal->terminal_coding)
|
||
#define FRAME_KEYBOARD_CODING(f) ((f)->terminal->keyboard_coding)
|
||
|
||
#define TERMINAL_TERMINAL_CODING(d) ((d)->terminal_coding)
|
||
#define TERMINAL_KEYBOARD_CODING(d) ((d)->keyboard_coding)
|
||
|
||
#define FRAME_RIF(f) ((f)->terminal->rif)
|
||
|
||
#define FRAME_TERMINAL(f) ((f)->terminal)
|
||
|
||
/* Return true if the terminal device is not suspended. */
|
||
#define TERMINAL_ACTIVE_P(d) \
|
||
(((d)->type != output_termcap && (d)->type != output_msdos_raw) \
|
||
|| (d)->display_info.tty->input)
|
||
|
||
/* Return font cache data for the specified terminal. The historical
|
||
name is grossly misleading, actually it is (NAME . FONT-LIST-CACHE). */
|
||
#if defined (HAVE_X_WINDOWS)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_x_window ? t->display_info.x->name_list_element : Qnil)
|
||
#elif defined (HAVE_NTGUI)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_w32 ? t->display_info.w32->name_list_element : Qnil)
|
||
#elif defined (HAVE_NS)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_ns ? t->display_info.ns->name_list_element : Qnil)
|
||
#elif defined (HAVE_PGTK)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_pgtk ? t->display_info.pgtk->name_list_element : Qnil)
|
||
#elif defined (HAVE_HAIKU)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_haiku ? t->display_info.haiku->name_list_element : Qnil)
|
||
#elif defined (HAVE_ANDROID)
|
||
#define TERMINAL_FONT_CACHE(t) \
|
||
(t->type == output_android ? t->display_info.android->name_list_element : Qnil)
|
||
#endif
|
||
|
||
extern struct terminal *decode_live_terminal (Lisp_Object);
|
||
extern struct terminal *decode_tty_terminal (Lisp_Object);
|
||
extern struct terminal *get_named_terminal (const char *);
|
||
extern struct terminal *create_terminal (enum output_method,
|
||
struct redisplay_interface *);
|
||
extern void delete_terminal (struct terminal *);
|
||
extern void delete_terminal_internal (struct terminal *);
|
||
extern Lisp_Object terminal_glyph_code (struct terminal *, int);
|
||
|
||
/* The initial terminal device, created by initial_term_init. */
|
||
extern struct terminal *initial_terminal;
|
||
|
||
extern unsigned char *encode_terminal_code (struct glyph *, int,
|
||
struct coding_system *);
|
||
|
||
#ifdef HAVE_GPM
|
||
extern void close_gpm (int gpm_fd);
|
||
#endif
|
||
|
||
#ifdef WINDOWSNT
|
||
extern int cursorX (struct tty_display_info *);
|
||
extern int cursorY (struct tty_display_info *);
|
||
#else
|
||
#define cursorX(t) curX(t)
|
||
#define cursorY(t) curY(t)
|
||
#endif
|
||
|
||
INLINE_HEADER_END
|
||
|
||
#endif /* EMACS_TERMHOOKS_H */
|