mirror of
https://git.FreeBSD.org/ports.git
synced 2024-12-01 01:17:02 +00:00
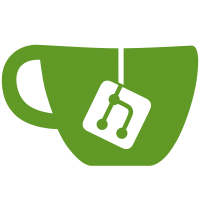
adds some features, there is at least one crasher I've hit so far but I don't know if it is anomoly or not yet. Approved by: kris
172 lines
5.6 KiB
Bash
172 lines
5.6 KiB
Bash
#!/bin/sh
|
|
# -*-shell-script-*-
|
|
#
|
|
# mkdistfile -- maintainer's utility to make a phoenix distfile
|
|
#
|
|
# Copyright (c) 2002, Alan Eldridge
|
|
# All rights reserved.
|
|
#
|
|
# Redistribution and use in source and binary forms, with or without
|
|
# modification, are permitted provided that the following conditions
|
|
# are met:
|
|
#
|
|
# * Redistributions of source code must retain the above copyright
|
|
# notice, this list of conditions and the following disclaimer.
|
|
#
|
|
# * Redistributions in binary form must reproduce the above copyright
|
|
# notice, this list of conditions and the following disclaimer in the
|
|
# documentation and/or other materials provided with the distribution.
|
|
#
|
|
# * Neither the name of the copyright owner nor the names of its
|
|
# contributors may be used to endorse or promote products derived
|
|
# from this software without specific prior written permission.
|
|
#
|
|
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
|
|
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
|
|
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
|
|
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
|
|
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
|
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
|
|
# POSSIBILITY OF SUCH DAMAGE.
|
|
#
|
|
# $FreeBSD$
|
|
#
|
|
# 2002/10/21 alane@geeksrus.net
|
|
#
|
|
|
|
ORIGDIR=$(/bin/pwd); export ORIGDIR
|
|
APPNAME=${0##*/};APPNAME=${APPNAME#-}; export APPNAME
|
|
test -z "$TMPDIR" && TMPDIR=/tmp; export TMPDIR
|
|
test -z "$HOSTNAME" && HOSTNAME=$(hostname); export HOSTNAME
|
|
warn() { echo "[$APPNAME:$$]" "****" "$@";}
|
|
status() { echo "[$APPNAME:$$]" "===>" "$@";}
|
|
error() { echo "[$APPNAME:$$]" "ERROR:" "$@";}
|
|
die() { echo "[$APPNAME:$$]" "FATAL:" "$@"; exit 1;}
|
|
unset echo_n; test "X`echo -n`" = "X-n" \
|
|
&& echo_n() { echo "$@\c"; } || echo_n() { echo -n "$@"; }
|
|
status_n() { echo_n "[$APPNAME:$$]" "===>" "$@";}
|
|
log() { local cmd=$1;shift;echo_n $(date "+%Y%m%d.%T") "";$cmd "$@";}
|
|
qexpr() { expr "$@" >/dev/null 2>&1;}
|
|
qw() { echo \""$@"\"; }
|
|
listfiles() { ls -1 ${1:+"$@"} 2>/dev/null; }
|
|
whichre() { local n=$#; local s="$1"; shift; while test $# -ge 1; do
|
|
qexpr "$s" : "$1"&& echo $(($n - $#)) && return 0; shift; done;
|
|
echo 0; return 1;}
|
|
matchre() { test $(whichre "$@") -gt 0; }
|
|
streq() { local s="$1"; shift; while test $# -ge 1; do
|
|
test "X$s" = "X$1" && return 0; shift; done; return 1;}
|
|
strupper() {
|
|
echo "$@"|tr abcdefghijklmnopqrstuvwxyz ABCDEFGHIJKLMNOPQRSTUVWXYZ
|
|
}
|
|
strlower() {
|
|
echo "$@"|tr ABCDEFGHIJKLMNOPQRSTUVWXYZ abcdefghijklmnopqrstuvwxyz
|
|
}
|
|
chdir() { cd "$1" || die cd $(qw $1) failed; }
|
|
absdir() { chdir "$1" && pwd; }
|
|
abspath() { local p=''; case "$1" in /*);; *)p="$(pwd)/";; esac; echo "$p$1"; }
|
|
tmpfile() { mktemp -t .$APPNAME ${1:+"$@"}; }
|
|
############################################################
|
|
# show usage and exit
|
|
############################################################
|
|
usage() {
|
|
cat <<EOF
|
|
Usage: $APPNAME [options] [--] rev[.yyyymmdd]
|
|
Options:
|
|
-h,--help Show this help.
|
|
-V,--version Show version number.
|
|
-v,--verbose Produce more verbose output.
|
|
-x,--debug Turn on shell command tracing.
|
|
-- Stop option processing.
|
|
EOF
|
|
exit $1
|
|
}
|
|
############################################################
|
|
# show version and exit
|
|
############################################################
|
|
VERSION='$Revision$'
|
|
VERSION=${VERSION#* }; VERSION=${VERSION% *}
|
|
version() { echo "$APPNAME $VERSION" \
|
|
"Copyright (c) 2002 Alan Eldridge"; exit $1;}
|
|
############################################################
|
|
# set default vars here
|
|
############################################################
|
|
DEBUG=0
|
|
VERBOSE=0; VFLG=''
|
|
DATETIME=$(date +%Y%m%d.%H%M)
|
|
DATE=${DATETIME%.*}
|
|
TIME=${DATETIME#*.}
|
|
############################################################
|
|
# do command line options
|
|
############################################################
|
|
while test $# -gt 0; do
|
|
n=1; case "$1" in
|
|
-h|--help) usage 0;;
|
|
-V|--version) version 0;;
|
|
-v|--verbose) VERBOSE=1;VFLG='-v';;
|
|
-x|--debug) set -x;DEBUG=1;;
|
|
--) shift; break;; -*) usage 1;; *) break;;
|
|
esac; shift $n
|
|
done
|
|
|
|
############################################################
|
|
# main(): script execution begins here
|
|
############################################################
|
|
|
|
test $# -eq 1 || usage 1
|
|
|
|
REV=$1
|
|
test ${REV##*.} = today && REV=${REV%.*}.$DATE
|
|
|
|
status "pruning tree..."
|
|
exfile=$(tmpfile)
|
|
cat >>$exfile <<'EOF'
|
|
^.*/CVS($|/)
|
|
^.*/macbuild($|/)
|
|
^.*/package($|/)
|
|
^.*/.cvsignore($|/)
|
|
^.*/windows($|/)
|
|
^.*/activex($|/)
|
|
^.*/os2($|/)
|
|
^.*/solaris($|/)
|
|
^.*/gc($|/)
|
|
^mozilla/apache($|/)
|
|
^mozilla/calendar($|/)
|
|
^mozilla/cck($|/)
|
|
^mozilla/chimera($|/)
|
|
^mozilla/ef($|/)
|
|
^mozilla/embed.mak($|/)
|
|
^mozilla/embed.mk($|/)
|
|
^mozilla/gconfig($|/)
|
|
^mozilla/gfx2($|/)
|
|
^mozilla/grendel($|/)
|
|
^mozilla/java($|/)
|
|
^mozilla/js2($|/)
|
|
^mozilla/mail($|/)
|
|
^mozilla/mailnews($|/)
|
|
^mozilla/mozilla($|/)
|
|
^mozilla/mozilla.kdevprj($|/)
|
|
^mozilla/mozilla.lsm($|/)
|
|
^mozilla/msgsdk($|/)
|
|
^mozilla/mstone($|/)
|
|
^mozilla/nglayout.mac($|/)
|
|
^mozilla/nunet($|/)
|
|
^mozilla/other-licenses($|/)
|
|
^mozilla/privacy($|/)
|
|
^mozilla/silentdl($|/)
|
|
^mozilla/timer($|/)
|
|
^mozilla/trex.mak($|/)
|
|
^mozilla/trex.mk($|/)
|
|
^mozilla/webtools($|/)
|
|
EOF
|
|
test -d mozilla || die "No mozilla dir here."
|
|
find mozilla 2>/dev/null | egrep -f $exfile | xargs rm -fr
|
|
rm -f $exfile
|
|
status "making phoenix-$REV.tar.bz2 ..."
|
|
tar -cjpf phoenix-$REV.tar.bz2 mozilla
|
|
status "done."
|
|
#EOF
|