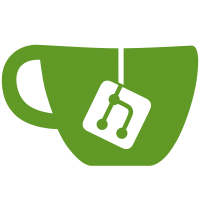
I am going to start parsing fields out of the json event objects, so it makes sense to give each event its own type to avoid the verbosity of parsing the values out of the json object every time they are needed.
24 lines
1.0 KiB
Rust
24 lines
1.0 KiB
Rust
use serde_json::{self, Map};
|
|
|
|
pub fn get_json_object(obj: &serde_json::Value) -> &Map<String, serde_json::Value> {
|
|
match obj {
|
|
serde_json::Value::Null => panic!("Unexpected json type"),
|
|
serde_json::Value::Bool(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::Number(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::String(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::Array(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::Object(event_object) => event_object,
|
|
}
|
|
}
|
|
|
|
pub fn get_json_string(str: &serde_json::Value) -> &String {
|
|
match str {
|
|
serde_json::Value::Null => panic!("Unexpected json type"),
|
|
serde_json::Value::Bool(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::Number(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::String(txt) => txt,
|
|
serde_json::Value::Array(_) => panic!("Unexpected json type"),
|
|
serde_json::Value::Object(_) => panic!("Unexpected json type"),
|
|
}
|
|
}
|