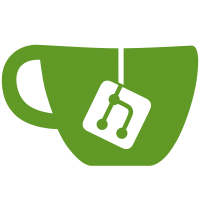
This is largely to make changing the type signature of these functions easier by significantly reducing the amount of places that duplicates the signature.
38 lines
910 B
Rust
38 lines
910 B
Rust
use std::path::Path;
|
|
|
|
use serde::Serialize;
|
|
|
|
use crate::config::Config;
|
|
use crate::error::CustomError;
|
|
use crate::intermediate::IDocumentElement;
|
|
|
|
use super::macros::render;
|
|
use super::RenderHeading;
|
|
use super::RenderSection;
|
|
|
|
#[derive(Debug, Serialize)]
|
|
#[serde(untagged)]
|
|
pub(crate) enum RenderDocumentElement {
|
|
Heading(RenderHeading),
|
|
Section(RenderSection),
|
|
}
|
|
|
|
render!(
|
|
RenderDocumentElement,
|
|
IDocumentElement,
|
|
original,
|
|
config,
|
|
output_directory,
|
|
output_file,
|
|
{
|
|
match original {
|
|
IDocumentElement::Heading(inner) => Ok(RenderDocumentElement::Heading(
|
|
RenderHeading::new(config, output_directory, output_file, inner)?,
|
|
)),
|
|
IDocumentElement::Section(inner) => Ok(RenderDocumentElement::Section(
|
|
RenderSection::new(config, output_directory, output_file, inner)?,
|
|
)),
|
|
}
|
|
}
|
|
);
|