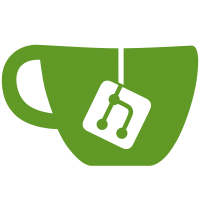
The issue is plain text is eating the line break so paragraph is failing since it expects a line break at the end.
53 lines
1.2 KiB
Rust
53 lines
1.2 KiB
Rust
use nom::combinator::map;
|
|
use nom::combinator::not;
|
|
|
|
use super::error::Res;
|
|
use super::parser_with_context::parser_with_context;
|
|
use super::plain_text::plain_text;
|
|
use super::source::Source;
|
|
use super::Context;
|
|
|
|
#[derive(Debug)]
|
|
pub enum Object<'s> {
|
|
TextMarkup(TextMarkup<'s>),
|
|
PlainText(PlainText<'s>),
|
|
RegularLink(RegularLink<'s>),
|
|
}
|
|
|
|
#[derive(Debug)]
|
|
pub struct TextMarkup<'s> {
|
|
pub source: &'s str,
|
|
}
|
|
|
|
#[derive(Debug)]
|
|
pub struct PlainText<'s> {
|
|
pub source: &'s str,
|
|
}
|
|
|
|
#[derive(Debug)]
|
|
pub struct RegularLink<'s> {
|
|
pub source: &'s str,
|
|
}
|
|
|
|
impl<'s> Source<'s> for Object<'s> {
|
|
fn get_source(&'s self) -> &'s str {
|
|
match self {
|
|
Object::TextMarkup(obj) => obj.source,
|
|
Object::PlainText(obj) => obj.source,
|
|
Object::RegularLink(obj) => obj.source,
|
|
}
|
|
}
|
|
}
|
|
|
|
#[tracing::instrument(ret, level = "debug")]
|
|
pub fn standard_set_object<'r, 's>(
|
|
context: Context<'r, 's>,
|
|
input: &'s str,
|
|
) -> Res<&'s str, Object<'s>> {
|
|
not(|i| context.check_exit_matcher(i))(input)?;
|
|
|
|
let plain_text_matcher = parser_with_context!(plain_text)(context);
|
|
|
|
map(plain_text_matcher, Object::PlainText)(input)
|
|
}
|