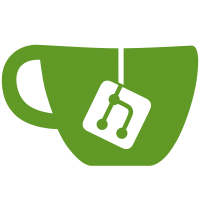
The parsers are the most complicated part, so I want them in their own files. I am uncertain if I want to move their corresponding structs or just the parsers.
34 lines
895 B
Rust
34 lines
895 B
Rust
use crate::parser::parser_with_context::parser_with_context;
|
|
use nom::combinator::map;
|
|
use nom::combinator::not;
|
|
|
|
use super::error::Res;
|
|
use super::greater_element::PlainList;
|
|
use super::lesser_element::Paragraph;
|
|
use super::paragraph::paragraph;
|
|
use super::source::Source;
|
|
use super::Context;
|
|
|
|
#[derive(Debug)]
|
|
pub enum Element<'s> {
|
|
Paragraph(Paragraph<'s>),
|
|
PlainList(PlainList<'s>),
|
|
}
|
|
|
|
impl<'s> Source<'s> for Element<'s> {
|
|
fn get_source(&'s self) -> &'s str {
|
|
match self {
|
|
Element::Paragraph(obj) => obj.source,
|
|
Element::PlainList(obj) => obj.source,
|
|
}
|
|
}
|
|
}
|
|
|
|
pub fn element<'r, 's>(context: Context<'r, 's>, input: &'s str) -> Res<&'s str, Element<'s>> {
|
|
not(|i| context.check_exit_matcher(i))(input)?;
|
|
|
|
let paragraph_matcher = parser_with_context!(paragraph)(context);
|
|
|
|
map(paragraph_matcher, Element::Paragraph)(input)
|
|
}
|