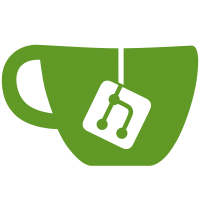
This ensures the parsers can take into account the affiliated keywords when setting their source without needing the SetSource trait.
188 lines
6.4 KiB
Rust
188 lines
6.4 KiB
Rust
use nom::branch::alt;
|
|
use nom::bytes::complete::is_not;
|
|
use nom::bytes::complete::tag;
|
|
use nom::character::complete::line_ending;
|
|
use nom::character::complete::space0;
|
|
use nom::combinator::not;
|
|
use nom::combinator::opt;
|
|
use nom::combinator::peek;
|
|
use nom::combinator::recognize;
|
|
use nom::combinator::verify;
|
|
use nom::multi::many0;
|
|
use nom::multi::many1;
|
|
use nom::multi::many_till;
|
|
use nom::sequence::tuple;
|
|
|
|
use super::keyword::affiliated_keyword;
|
|
use super::keyword::table_formula_keyword;
|
|
use super::object_parser::table_cell_set_object;
|
|
use super::org_source::OrgSource;
|
|
use super::util::exit_matcher_parser;
|
|
use super::util::get_name;
|
|
use super::util::maybe_consume_trailing_whitespace_if_not_exiting;
|
|
use super::util::org_line_ending;
|
|
use crate::context::parser_with_context;
|
|
use crate::context::ContextElement;
|
|
use crate::context::ExitClass;
|
|
use crate::context::ExitMatcherNode;
|
|
use crate::context::RefContext;
|
|
use crate::error::Res;
|
|
use crate::parser::util::get_consumed;
|
|
use crate::parser::util::start_of_line;
|
|
use crate::types::Table;
|
|
use crate::types::TableCell;
|
|
use crate::types::TableRow;
|
|
|
|
/// Parse an org-mode-style table
|
|
///
|
|
/// This is not the table.el style.
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
pub(crate) fn org_mode_table<'b, 'g, 'r, 's>(
|
|
context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, Table<'s>> {
|
|
let (remaining, affiliated_keywords) = many0(affiliated_keyword)(input)?;
|
|
start_of_line(remaining)?;
|
|
peek(tuple((space0, tag("|"))))(remaining)?;
|
|
|
|
let contexts = [
|
|
ContextElement::ConsumeTrailingWhitespace(true),
|
|
ContextElement::Context("table"),
|
|
ContextElement::ExitMatcherNode(ExitMatcherNode {
|
|
class: ExitClass::Alpha,
|
|
exit_matcher: &table_end,
|
|
}),
|
|
];
|
|
let parser_context = context.with_additional_node(&contexts[0]);
|
|
let parser_context = parser_context.with_additional_node(&contexts[1]);
|
|
let parser_context = parser_context.with_additional_node(&contexts[2]);
|
|
|
|
let org_mode_table_row_matcher = parser_with_context!(org_mode_table_row)(&parser_context);
|
|
let exit_matcher = parser_with_context!(exit_matcher_parser)(&parser_context);
|
|
|
|
let (remaining, (children, _exit_contents)) =
|
|
many_till(org_mode_table_row_matcher, exit_matcher)(remaining)?;
|
|
|
|
let (remaining, formulas) =
|
|
many0(parser_with_context!(table_formula_keyword)(context))(remaining)?;
|
|
|
|
let (remaining, _trailing_ws) =
|
|
maybe_consume_trailing_whitespace_if_not_exiting(context, remaining)?;
|
|
let source = get_consumed(input, remaining);
|
|
|
|
Ok((
|
|
remaining,
|
|
Table {
|
|
source: source.into(),
|
|
name: get_name(&affiliated_keywords),
|
|
formulas,
|
|
children,
|
|
},
|
|
))
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
pub(crate) fn detect_table<'s>(input: OrgSource<'s>) -> Res<OrgSource<'s>, ()> {
|
|
let (input, _) = many0(affiliated_keyword)(input)?;
|
|
tuple((start_of_line, space0, tag("|")))(input)?;
|
|
Ok((input, ()))
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn table_end<'b, 'g, 'r, 's>(
|
|
_context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, OrgSource<'s>> {
|
|
start_of_line(input)?;
|
|
recognize(tuple((space0, not(tag("|")))))(input)
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn org_mode_table_row<'b, 'g, 'r, 's>(
|
|
context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, TableRow<'s>> {
|
|
alt((
|
|
parser_with_context!(org_mode_table_row_rule)(context),
|
|
parser_with_context!(org_mode_table_row_regular)(context),
|
|
))(input)
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn org_mode_table_row_rule<'b, 'g, 'r, 's>(
|
|
_context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, TableRow<'s>> {
|
|
start_of_line(input)?;
|
|
let (remaining, _) = tuple((space0, tag("|-"), opt(is_not("\r\n")), org_line_ending))(input)?;
|
|
let source = get_consumed(input, remaining);
|
|
Ok((
|
|
remaining,
|
|
TableRow {
|
|
source: source.into(),
|
|
children: Vec::new(),
|
|
},
|
|
))
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn org_mode_table_row_regular<'b, 'g, 'r, 's>(
|
|
context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, TableRow<'s>> {
|
|
start_of_line(input)?;
|
|
let (remaining, _) = tuple((space0, tag("|")))(input)?;
|
|
let (remaining, children) =
|
|
many1(parser_with_context!(org_mode_table_cell)(context))(remaining)?;
|
|
let (remaining, _tail) = recognize(tuple((space0, org_line_ending)))(remaining)?;
|
|
let source = get_consumed(input, remaining);
|
|
Ok((
|
|
remaining,
|
|
TableRow {
|
|
source: source.into(),
|
|
children,
|
|
},
|
|
))
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn org_mode_table_cell<'b, 'g, 'r, 's>(
|
|
context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, TableCell<'s>> {
|
|
let parser_context = ContextElement::ExitMatcherNode(ExitMatcherNode {
|
|
class: ExitClass::Beta,
|
|
exit_matcher: &org_mode_table_cell_end,
|
|
});
|
|
let parser_context = context.with_additional_node(&parser_context);
|
|
let table_cell_set_object_matcher =
|
|
parser_with_context!(table_cell_set_object)(&parser_context);
|
|
let exit_matcher = parser_with_context!(exit_matcher_parser)(&parser_context);
|
|
let (remaining, (children, _exit_contents)) = verify(
|
|
many_till(table_cell_set_object_matcher, exit_matcher),
|
|
|(children, exit_contents)| {
|
|
!children.is_empty() || Into::<&str>::into(exit_contents).ends_with("|")
|
|
},
|
|
)(input)?;
|
|
|
|
let (remaining, _tail) = org_mode_table_cell_end(&parser_context, remaining)?;
|
|
|
|
let source = get_consumed(input, remaining);
|
|
|
|
Ok((
|
|
remaining,
|
|
TableCell {
|
|
source: source.into(),
|
|
children,
|
|
},
|
|
))
|
|
}
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
fn org_mode_table_cell_end<'b, 'g, 'r, 's>(
|
|
_context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, OrgSource<'s>> {
|
|
recognize(tuple((space0, alt((tag("|"), peek(line_ending))))))(input)
|
|
}
|