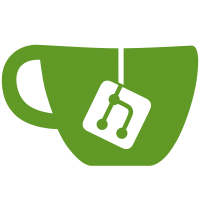
This prevents context tree from deeper in the parse impacting exit checks for earlier in the parse. This is needed due to the trailing whitespace context element.
179 lines
4.3 KiB
Rust
179 lines
4.3 KiB
Rust
use std::fmt::Debug;
|
|
use std::rc::Rc;
|
|
|
|
#[derive(Debug)]
|
|
pub struct List<T> {
|
|
head: Option<Rc<Node<T>>>,
|
|
}
|
|
|
|
impl<T> Clone for List<T> {
|
|
fn clone(&self) -> Self {
|
|
List {
|
|
head: self.head.clone(),
|
|
}
|
|
}
|
|
}
|
|
|
|
#[derive(Debug, Clone)]
|
|
pub struct Node<T> {
|
|
data: T,
|
|
parent: Option<Rc<Node<T>>>,
|
|
}
|
|
|
|
impl<T> Node<T> {
|
|
pub fn get_data(&self) -> &T {
|
|
&self.data
|
|
}
|
|
}
|
|
|
|
impl<T> List<T> {
|
|
pub fn new() -> Self {
|
|
List { head: None }
|
|
}
|
|
|
|
pub fn branch_from(trunk: &Rc<Node<T>>) -> Self {
|
|
List {
|
|
head: Some(trunk.clone()),
|
|
}
|
|
}
|
|
|
|
pub fn push_front(&self, data: T) -> List<T> {
|
|
List {
|
|
head: Some(Rc::new(Node {
|
|
data: data,
|
|
parent: self.head.clone(),
|
|
})),
|
|
}
|
|
}
|
|
|
|
pub fn pop_front(&mut self) -> (Option<T>, List<T>) {
|
|
match self.head.take() {
|
|
None => (None, List::new()),
|
|
Some(popped_node) => {
|
|
let extracted_node = match Rc::try_unwrap(popped_node) {
|
|
Ok(node) => node,
|
|
Err(_) => panic!("try_unwrap failed on Rc in pop_front on List."),
|
|
};
|
|
(
|
|
Some(extracted_node.data),
|
|
List {
|
|
head: extracted_node.parent,
|
|
},
|
|
)
|
|
}
|
|
}
|
|
}
|
|
|
|
pub fn without_front(&self) -> List<T> {
|
|
List {
|
|
head: self.head.as_ref().map(|node| node.parent.clone()).flatten(),
|
|
}
|
|
}
|
|
|
|
pub fn get_data(&self) -> Option<&T> {
|
|
self.head.as_ref().map(|rc_node| &rc_node.data)
|
|
}
|
|
|
|
pub fn is_empty(&self) -> bool {
|
|
self.head.is_none()
|
|
}
|
|
|
|
pub fn ptr_eq(&self, other: &List<T>) -> bool {
|
|
match (self.head.as_ref(), other.head.as_ref()) {
|
|
(None, None) => true,
|
|
(None, Some(_)) | (Some(_), None) => false,
|
|
(Some(me), Some(them)) => Rc::ptr_eq(me, them),
|
|
}
|
|
}
|
|
|
|
pub fn iter(&self) -> impl Iterator<Item = &Rc<Node<T>>> {
|
|
NodeIter {
|
|
position: &self.head,
|
|
}
|
|
}
|
|
|
|
pub fn iter_until<'a>(&'a self, other: &'a List<T>) -> impl Iterator<Item = &Rc<Node<T>>> {
|
|
NodeIterUntil {
|
|
position: &self.head,
|
|
stop: &other.head,
|
|
}
|
|
}
|
|
|
|
pub fn into_iter_until<'a>(self, other: &'a List<T>) -> impl Iterator<Item = T> + 'a {
|
|
NodeIntoIterUntil {
|
|
position: self,
|
|
stop: &other,
|
|
}
|
|
}
|
|
}
|
|
|
|
pub struct NodeIter<'a, T> {
|
|
position: &'a Option<Rc<Node<T>>>,
|
|
}
|
|
|
|
impl<'a, T> Iterator for NodeIter<'a, T> {
|
|
type Item = &'a Rc<Node<T>>;
|
|
|
|
fn next(&mut self) -> Option<Self::Item> {
|
|
let (return_value, next_position) = match &self.position {
|
|
None => return None,
|
|
Some(rc_node) => {
|
|
let next_position = &rc_node.parent;
|
|
let return_value = rc_node;
|
|
(return_value, next_position)
|
|
}
|
|
};
|
|
self.position = next_position;
|
|
Some(return_value)
|
|
}
|
|
}
|
|
|
|
pub struct NodeIterUntil<'a, T> {
|
|
position: &'a Option<Rc<Node<T>>>,
|
|
stop: &'a Option<Rc<Node<T>>>,
|
|
}
|
|
|
|
impl<'a, T> Iterator for NodeIterUntil<'a, T> {
|
|
type Item = &'a Rc<Node<T>>;
|
|
|
|
fn next(&mut self) -> Option<Self::Item> {
|
|
match (self.position, self.stop) {
|
|
(_, None) => {}
|
|
(None, _) => {}
|
|
(Some(this_rc), Some(stop_rc)) => {
|
|
if Rc::ptr_eq(this_rc, stop_rc) {
|
|
return None;
|
|
}
|
|
}
|
|
};
|
|
let (return_value, next_position) = match &self.position {
|
|
None => return None,
|
|
Some(rc_node) => {
|
|
let next_position = &rc_node.parent;
|
|
let return_value = rc_node;
|
|
(return_value, next_position)
|
|
}
|
|
};
|
|
self.position = next_position;
|
|
Some(return_value)
|
|
}
|
|
}
|
|
|
|
pub struct NodeIntoIterUntil<'a, T> {
|
|
position: List<T>,
|
|
stop: &'a List<T>,
|
|
}
|
|
|
|
impl<'a, T> Iterator for NodeIntoIterUntil<'a, T> {
|
|
type Item = T;
|
|
|
|
fn next(&mut self) -> Option<Self::Item> {
|
|
if self.position.ptr_eq(self.stop) {
|
|
return None;
|
|
}
|
|
let (popped_element, new_position) = self.position.pop_front();
|
|
self.position = new_position;
|
|
popped_element
|
|
}
|
|
}
|