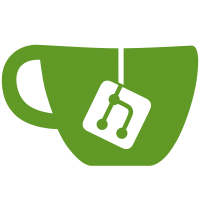
This might be used for look-behind instead of storing previous element nodes in the context tree.
26 lines
891 B
Rust
26 lines
891 B
Rust
use super::parser_context::ContextElement;
|
|
use super::Context;
|
|
|
|
/// Check if we are below a section of the given section type regardless of depth
|
|
pub fn in_section<'r, 's, 'x>(context: Context<'r, 's>, section_name: &'x str) -> bool {
|
|
for thing in context.iter() {
|
|
match thing.get_data() {
|
|
ContextElement::Context(name) if *name == section_name => return true,
|
|
_ => {}
|
|
}
|
|
}
|
|
false
|
|
}
|
|
|
|
/// Checks if we are currently an immediate child of the given section type
|
|
pub fn immediate_in_section<'r, 's, 'x>(context: Context<'r, 's>, section_name: &'x str) -> bool {
|
|
for thing in context.iter() {
|
|
match thing.get_data() {
|
|
ContextElement::Context(name) if *name == section_name => return true,
|
|
ContextElement::Context(name) if *name != section_name => return false,
|
|
_ => {}
|
|
}
|
|
}
|
|
false
|
|
}
|