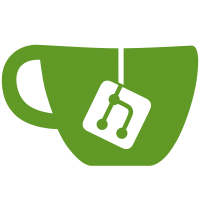
This ensures the parsers can take into account the affiliated keywords when setting their source without needing the SetSource trait.
46 lines
1.5 KiB
Rust
46 lines
1.5 KiB
Rust
use nom::branch::alt;
|
|
use nom::bytes::complete::tag;
|
|
use nom::character::complete::line_ending;
|
|
use nom::character::complete::space0;
|
|
use nom::combinator::eof;
|
|
use nom::combinator::recognize;
|
|
use nom::combinator::verify;
|
|
use nom::multi::many0;
|
|
use nom::multi::many1_count;
|
|
use nom::sequence::tuple;
|
|
|
|
use super::keyword::affiliated_keyword;
|
|
use super::org_source::OrgSource;
|
|
use super::util::get_consumed;
|
|
use super::util::get_name;
|
|
use super::util::maybe_consume_trailing_whitespace_if_not_exiting;
|
|
use crate::context::RefContext;
|
|
use crate::error::Res;
|
|
use crate::parser::util::start_of_line;
|
|
use crate::types::HorizontalRule;
|
|
|
|
#[cfg_attr(feature = "tracing", tracing::instrument(ret, level = "debug"))]
|
|
pub(crate) fn horizontal_rule<'b, 'g, 'r, 's>(
|
|
context: RefContext<'b, 'g, 'r, 's>,
|
|
input: OrgSource<'s>,
|
|
) -> Res<OrgSource<'s>, HorizontalRule<'s>> {
|
|
let (remaining, affiliated_keywords) = many0(affiliated_keyword)(input)?;
|
|
start_of_line(remaining)?;
|
|
let (remaining, _rule) = recognize(tuple((
|
|
space0,
|
|
verify(many1_count(tag("-")), |dashes| *dashes >= 5),
|
|
space0,
|
|
alt((line_ending, eof)),
|
|
)))(remaining)?;
|
|
let (remaining, _trailing_ws) =
|
|
maybe_consume_trailing_whitespace_if_not_exiting(context, remaining)?;
|
|
let source = get_consumed(input, remaining);
|
|
Ok((
|
|
remaining,
|
|
HorizontalRule {
|
|
source: source.into(),
|
|
name: get_name(&affiliated_keywords),
|
|
},
|
|
))
|
|
}
|